A point represents a single location in space and is the building block for all other geometry types. A point has, at a minimum, an x-coordinate and a y-coordinate. The coordinates of a point can be in linear units such as feet or meters, or they can be in angular units such as degrees or radians. The associated spatial reference specifies the units of the coordinates. In the case of a geographic coordinate system, the x-coordinate is the longitude and the y-coordinate is the latitude.
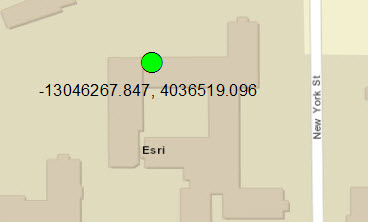
Boundary, Interior & Exterior
The boundary of a point is the empty set, the interior is the point itself, and the exterior is the set of points that are not in the interior. It is important to understand the boundary, interior and exterior of a geometry when using the various operators. The relational operators, for example, rely heavily on these concepts.
Valid points
Every point is valid.
A valid geometry is said to be simple. See the Simplify operator for a detailed specification of simple geometries.
JSON format
A point can be represented as a JSON string. A point in JSON format contains x
and y
fields and an optional spatialReference
. A point can also have m
and z
fields.
A point is empty when its x
field is present and has the value null
or the string "Nan".
An empty point has no location in space.
Syntax
{ "x": <x>, "y": <y>, "z": <z>, "m": <m>, "spatialReference" : {"wkid" : <wkid>} }
2D point
{ "x": 32462, "y": -57839, "spatialReference" : {"wkid" : 54004} }
3D point with Ms
{ "x": 32462, "y": -57839, "z": 20, "m": 1, "spatialReference" : {"wkid" : 54004} }
Empty point
{ "x": null }
{ "x": "NaN" }
Creating a point
To create a point, we can use the Point
class methods or one of the import operators.
Each of the code samples below creates a point which represents the summit of Mount Elbert, the highest
mountain in the Rocky Mountains.
The point looks like this on a map:
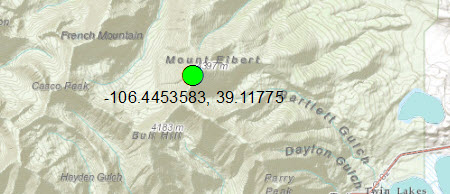
Point
class methods
We create a new Point
and set the xy-coordinates simultaneously in the constructor.
static Point createPoint1() { Point pt = new Point(-106.4453583, 39.11775); return pt; }
Import from JSON
We first create the JSON string which represents the point. We then call the execute
method of OperatorImportFromJson
.
static Point createPointFromJson() throws JsonParseException, IOException { String jsonString = "{\"x\":-106.4453583,\"y\":39.11775,\"spatialReference\":{\"wkid\":4326}}"; MapGeometry mapGeom = OperatorImportFromJson.local().execute(Geometry.Type.Point, jsonString); return (Point)mapGeom.getGeometry(); }
Import from GeoJSON
We first create the GeoJSON string which represents the point. We then call the execute
method of OperatorImportFromGeoJson
.
static Point createPointFromGeoJson() throws JsonParseException, IOException { String geoJsonString = "{\"type\":\"Point\",\"coordinates\":[-106.4453583,39.11775],\"crs\":\"EPSG:4326\"}"; MapGeometry mapGeom = OperatorImportFromGeoJson.local().execute(GeoJsonImportFlags.geoJsonImportDefaults, Geometry.Type.Point, geoJsonString, null); return (Point)mapGeom.getGeometry(); }
Import from WKT
We first create the WKT string which represents the point. We then call the execute
method of OperatorImportFromWkt
.
static Point createPointFromWKT() throws JsonParseException, IOException { String wktString = "Point (-106.4453583 39.11775)"; Geometry geom = OperatorImportFromWkt.local().execute(WktImportFlags.wktImportDefaults, Geometry.Type.Point, wktString, null); return (Point)geom; }