A multipoint is an ordered collection of points.
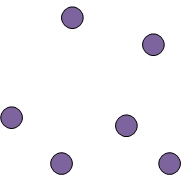
Boundary, Interior & Exterior
The boundary of a multipoint is the empty set, the interior is the set of points in the collection, and the exterior is the set of points that are not in the interior. It is important to understand the boundary, interior and exterior of a geometry when using the various operators. The relational operators, for example, rely heavily on these concepts.
Valid multipoints
A valid multipoint is such that every point in the collection is distinct. Tolerance is used to determine if two points are distinct. In other words, if the distance between each of the coordinates of two points is less than the tolerance specified by the spatial reference, then the points are considered equal.
For example, consider the multipoint shown below.
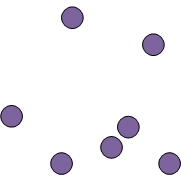
Is it a valid multipoint? It depends on the spatial reference.
Suppose the spatial reference for this multipoint uses WGS_1984_UTM_Zone_31N coordinate system with the default tolerance of 0.001 meters. The two points that are in the area outlined by the rectangle look pretty close. Let's zoom in on those two points.
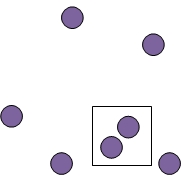
If the distance between the x-coordinates and the distance between the y-coordinates is less than 0.001 meters, then the multipoint is not a valid multipoint.
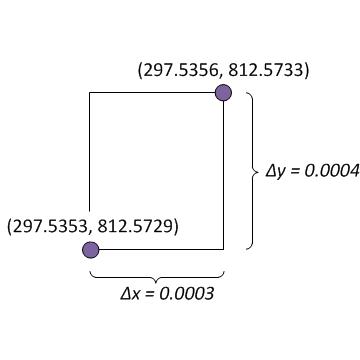
A valid multipoint is said to be simple. See the Simplify operator for a detailed specification of simple geometries.
Examples
Let's look at some examples of multipoints.
The example below shows six multipoints each of which represents major cities grouped by continent.
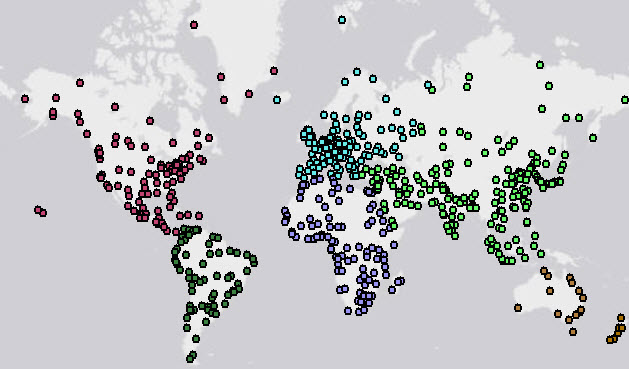
The next example shows four multipoints each of which represents major cities grouped by population.
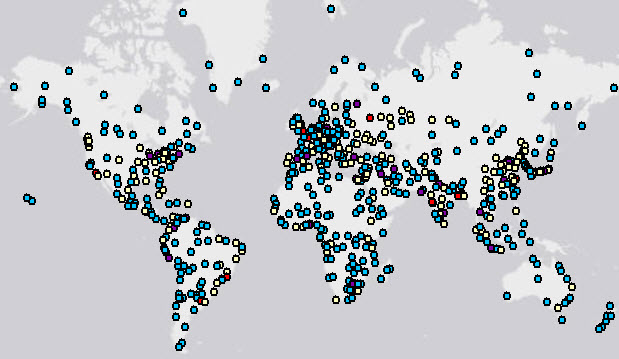
Here we have 13 multipoints each of which represents points of interest along possible routes for a road trip in the United States.
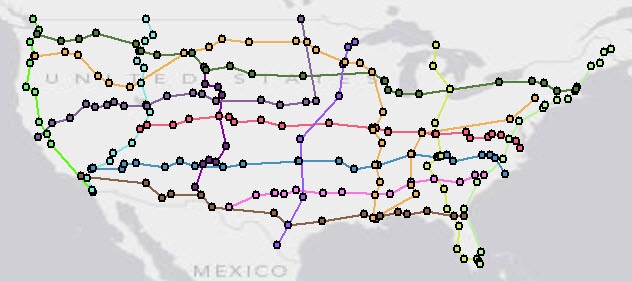
JSON format
A multipoint can be represented as a JSON string. A multipoint in JSON format contains an array of points
and an optional spatialReference
. A multipoint can also have boolean-valued hasM
and
hasZ
fields. The default value is false for both the hasM
and hasZ
fields.
Each element of the points
array is itself an array of 2, 3 or 4 numbers. It will have two
elements for 2D points, 3 elements for 3D points, 2 or 3 elements for 2D points with Ms and 3 or 4 elements for
3D points with Ms. In all cases, the x-coordinate is at index 0 of a point's array and the y-coordinate
is at index 1. For 2D points with Ms, the m-coordinate, if present, is at index 2. For 3D points, the
z-coordinate is required and is at index 2. For 3D points with Ms, the z-coordinate is at index 2 and
the m-coordinate, if present, is at index 3.
An empty multipoint is represented with an empty array for the points
field.
Syntax
{ "hasZ" : true | false, "hasM" : true | false, "points": [[<x1>,<y1>,<z1>,<m1>], ... ,[<xn>,<yn>,<zn>,<mn>]], "spatialReference" : {"wkid" : <wkid>} }
2D multipoint
{ "points": [[32462,-57839],[43892,-49160],[35637,-65035],[46009,-60379]], "spatialReference" : {"wkid" : 54004} }
3D multipoint with Ms
Note that the third point does not have a z-value, and the fourth point does not have an m-value.
{ "hasZ" : true, "hasM" : true, "points": [[32462,-57839,20,1],[43892,-49160,25,2],[35637,-65035,null,3],[46009,-60379,22]], "spatialReference" : {"wkid" : 54004} }
Empty multipoint
{"points": []}
Creating a multipoint
To create a multipoint, we can use the MultiPoint
class methods or one of the import operators.
Each of the code samples below creates a multipoint with six points.
The multipoint looks like this:
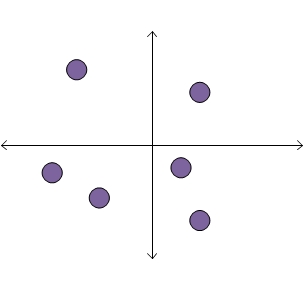
MultiPoint
class methods
We create a new MultiPoint
and add points by calling the add
method.
static MultiPoint createMultipoint1() {
MultiPoint mPoint = new MultiPoint();
// Add points
mPoint.add(1, 1);
mPoint.add(0.5, -0.5);
mPoint.add(1, -1.5);
mPoint.add(-1, -1);
mPoint.add(-2, -0.5);
mPoint.add(-1.5, 1.5);
return mPoint;
}
Import from JSON
We first create the JSON string which represents the multipoint. We then call the execute
method of OperatorImportFromJson
.
static MultiPoint createMultipointFromJson() throws JsonParseException, IOException { String jsonString = "{\"points\":[[1,1],[0.5,-0.5],[1,-1.5],[-1,-1],[-2,-0.5],[-1.5,1.5]]," + "\"spatialReference\":{\"wkid\":4326}}"; MapGeometry mapGeom = OperatorImportFromJson.local().execute(Geometry.Type.MultiPoint, jsonString); return (MultiPoint)mapGeom.getGeometry(); }
Import from GeoJSON
We first create the GeoJSON string which represents the multipoint. We then call the execute
method of OperatorImportFromGeoJson
.
static MultiPoint createMultipointFromGeoJson() throws JsonParseException, IOException { String geoJsonString = "{\"type\":\"MultiPoint\"," + "\"coordinates\":[[1,1],[0.5,-0.5],[1,-1.5],[-1,-1],[-2,-0.5],[-1.5,1.5]]," + "\"crs\":\"EPSG:4326\"}"; MapGeometry mapGeom = OperatorImportFromGeoJson.local().execute(GeoJsonImportFlags.geoJsonImportDefaults, Geometry.Type.MultiPoint, geoJsonString, null); return (MultiPoint)mapGeom.getGeometry(); }
Import from WKT
We first create the WKT string which represents the multipoint. We then call the execute
method of OperatorImportFromWkt
.
static MultiPoint createMultipointFromWKT() throws JsonParseException, IOException { String wktString = "MULTIPOINT ((1 1),(0.5 -0.5),(1 -1.5),(-1 -1),(-2 -0.5),(-1.5 1.5))"; Geometry geom = OperatorImportFromWkt.local().execute(WktImportFlags.wktImportDefaults, Geometry.Type.MultiPoint, wktString, null); return (MultiPoint)geom; }