An envelope is an axis-aligned rectanglular box of another geometry or a set of geometries. It is not equivalent to a rectangle polygon in certain operations.
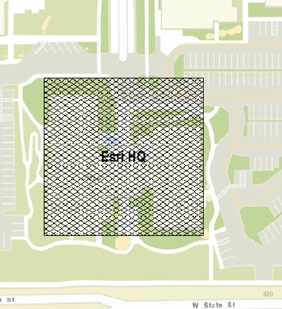
Envelope surrounding the Esri HQ
Valid Envelopes
All envelopes are valid since an envelope is a bounding box enclosing other geometry or set of geometries. The defining factor for an evelope is the fact that it is aligned to the axes. A 1-dimensional envelope is an envelope parallel to one of the axes while a 2-dimensional envelope is parallel to 2 axes.
Example
![]() | ![]() |
Polygon bounding a certain neighborhood | Envelope bounding all the geometries |
Envelope
class methods
In order to create an envelope, there are several methods defined in the Envelope
class which require a different set of arguments.
public void createEnvelope(){ /* One way to create an envelope is to specify the center point, * height and width of the envelope i.e. the region it is supposed to cover */ Point center = new Point(0, 0); double width = 20; double height = 20; Envelope env = new Envelope(center, width, height); /* Another way to create an envelope is to specify the X and Y extents. * xmin: Minimum X-coordinate, ymin: Minimum Y-coordinate, * xmax: Maximum X-coordinate and ymax: Maximum Y-coordinate */ Envelope env1 = new Envelope(0, 0, 30.5, 30.5); /* In order to create an envelope surrounding a point, * we specify the Point and its coordinates are used to set the extent of the envelope */ Envelope env2 = new Envelope(new Point(3.5, 4.4)); /* Loading an envelope using a JSON String */ MapGeometry mg = OperatorFactoryLocal.LoadGeometryFromJSONStringDbg( "{\"hasZ\":true,\"hasM\":true,\"xmin\":0,\"ymin\":0,\"zmin\":0,\"mmin\":null,\"xmax\":1,\"ymax\":1,\"zmax\":1,\"mmax\":null}"); Envelope e = (Envelope) mg.getGeometry(); }
Getting an envelope for a given geometry
We can query the envelope that is bound by a given geometry using the queryEnvelope(Envelope e)
method of the geometry's class.
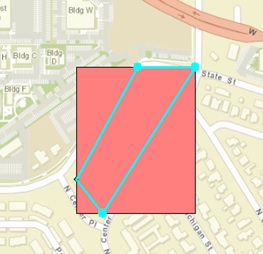
public void getGeometry(){
Polygon poly = new Polygon();
poly.startPath(-117.192322, 34.057129);
poly.lineTo(-117.191406, 34.057129);
poly.lineTo(-117.192871, 34.055298);
poly.lineTo(-117.193298, 34.055725);
Envelope env = new Envelope();
poly.queryEnvelope(env);
/* Now env contains the surrounding envelope for the specified polygon.
*/
}